1) Saves memory and reduces swapping. Many processes can use a single DLL simultaneously, sharing a single copy of the DLL in memory. In contrast, Windows must load a copy of the library code into memory for each application that is built with a static link library.
2) Saves disk space. Many applications can share a single copy of the DLL on disk. In contrast, each application built with a static link library has the library code linked into its executable image as a separate copy.
3) Upgrades to the DLL are easier. When the functions in a DLL change, the applications that use them do not need to be recompiled or relinked as long as the function arguments and return values do not change. In contrast, statically linked object code requires that the application be relinked when the functions change.
4) Provides after-market support. For example, a display driver DLL can be modified to support a display that was not available when the application was shipped.
5) Supports multi-language programs. Programs written in different programming languages can call the same DLL function as long as the programs follow the function's calling convention. The programs and the DLL function must be compatible in the following ways: the order in which the function expects its arguments to be pushed onto the stack, whether the function or the application is responsible for cleaning up the stack, and whether any arguments are passed in registers.
6)Eases the creation of international versions. By placing resources in a DLL, it is much easier to create international versions of an application. You can place the strings for each language version of your application in a separate resource DLL and have the different language versions load the appropriate resources.
A potential disadvantage to using DLLs is that the application is not self-contained; it depends on the existence of a separate DLL module.
With the use of dll's one application can use or perform multiple functionality by using multiple dll's. And another advantage is you dont have to give full code, just give applicxation .exe along with Library .dll and that 's enough to run the code.
To make an application by using dll, we need two different projects. One project will be Libarary for which we will make .dll and another will be appplication i.e. .exe file.
Ok let's start with Library project. Followings will be the steps:
1) Open visual studio and go to New Project. Choose "Win32 console project"
2) In application setting, choose DLL.
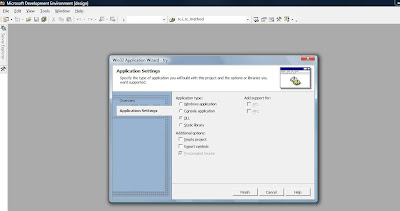
3) You will get 3 files in your project.
a) Dll_main.cpp b) stdafx.cpp and c) stdafx.h
you will also get some code already avaiable to those files. Just Keep it, those are required for compiler as an entry point to .dll
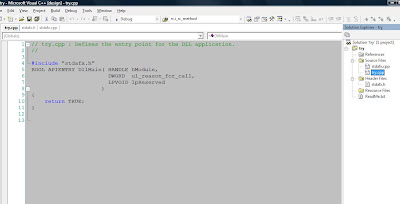
4) Now write your code or functions as usual only without main(). As main() will be in application project. Only thing is that the functions which will be avaialbe to applications, should to be exported. To export the function , you have to add "__declspec(dllexport)" before return type of function. I use define something like this
#define DLL_EXPORT __declspec(dllexport)
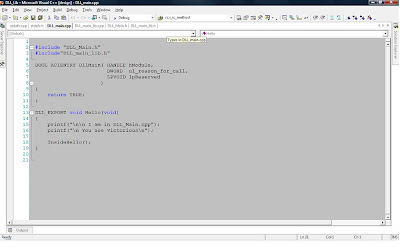
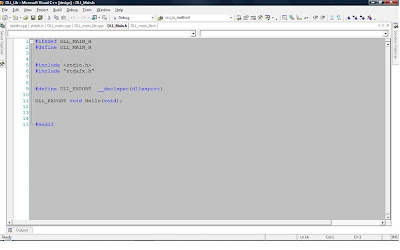
5) Other functions will be normal. no extra prefix. Just like I am using 'InsideHello()'in my DLL main.
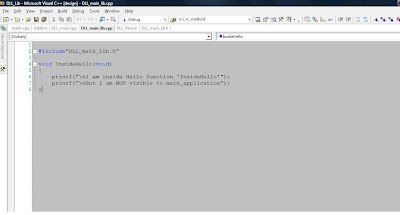
6) Now go to project porpoety page, and to C/C++ section , and set "Not Using Precompiled Headers"
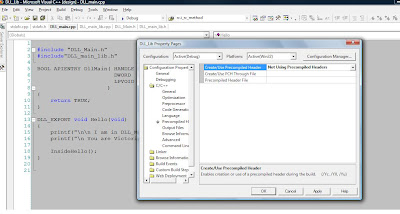
7) Now build the project, after successful build you will get ProjectName.lib and ProjectName.dll, so as for my case DLL_Lib.lib and DLL_Lib.dll. So you got your required .dll and .lib which we need for building application.
Now open new project for application. All settings will be normal as we do for usual .exe application.
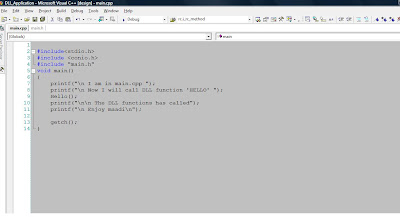
and exported function of DLL_lib should be imported here by adding "__declspec(dllimport) " before return type of those function.
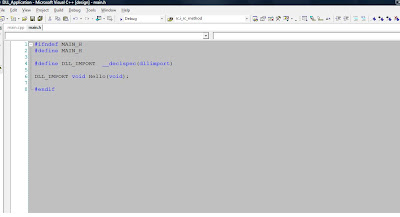
And now you have to add dependencies, for that go to property page and linker section and add as "input" the .llib file, for my case DLL_Lib.lib (which contain declarion of functions.)
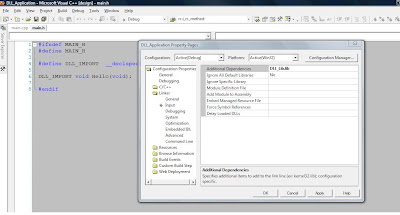
And copy the .lib and .dll from the Library project in the folder where .sln is present of application project. That's it, now build the project, if build successful i.e. your application has taken .lib and .dll and can be used. So now for test, keep .exe application and .dll of lib anywhere, it will run properly.
Now if you want handle totally different functionality, by only one application, make diffrerent .dll's and use there functions in your application. just like i was testing, two .dll in one exe.both written separately, but later thought to use in one application.
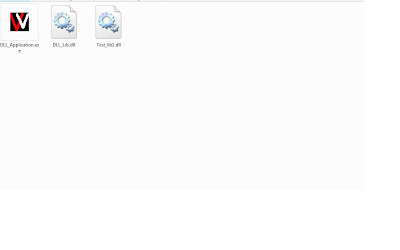
At this time it looks like Wordpress is the best blogging platform available right now.
ReplyDelete(from what I've read) Is that what you're
using on your blog?
My website - Marc Primo